Having got everything running and tested a Pacman-style sprite, I figure the next step is to learn how to draw backgrounds. I already know how to load an image in as the backdrop, but what about a grid-style background made of tiles?
The Playdate SDK has a Tilemap object, but not much sample code. Fortunately there’s a helpful discussion here where people have been working it out.
Using Arrays
First, arrays: I’m going to need to store each level as grid-like array of tile references. In Lua these are set up as tables, initialised as
anArray = {}
or with contents
someArray{1,2,3}
And then referenced as someArray[index]. The only weird thing is that these are 1-indexed, so someArray[2] = 2, not 3. This is.. going to hurt my brain.
To build a test level Tilemap I need some tile graphics, so I borrowed an image from one of the SDK examples:
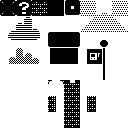
I can use the top row of that for some neat 16×16 placeholder tiles until I find a good pixel editor to make some of my own.
Using a Tilemap
In order to draw a tilemap on screen, you need to:
1) Initiate a Tilemap object and set its width and height (again, 1-indexed). My tiles are 16×16 pixels, so I’m drawing a 15 by 15 grid.
map = gfx.tilemap.new()
map:setSize(15,15)
2) Assign an imagetable, which is the image with the tiles in it. Note the size of the contained images, in the file name
spritesheet = gfx.imagetable.new("Images/bg-table-16-16.png")
assert( spritesheet )
map:setImageTable(spritesheet)
3) Use setTiles to apply an array of “for each cell in the map, which tile should be used” (15 is the width of my map):
map:setTiles(myLevel, 15)
In my case myLevel is just a 15*15 item long array, where each value is an index from 1 to 8 referencing the tiles in the top row of the graphic.
4) Finally draw it:
map:draw(80,0) -- draw the map in the middle of the display
-- test updating one item in the map
map:setTileAtPosition(15, 15, 1)
The result: a 15×15 level map, made up of tiles, in the middle of the screen:
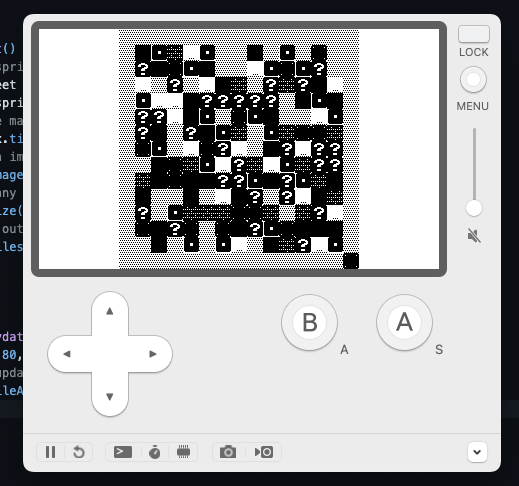
Note that I set my myLevel to roughly draw a grey box around the outside of the 15×15 map, and then had random items dropped in every other element. And then finally the last square (15×15) is updated using the setTileAtPosition, just to test that.
Full code here.