Having worked out how to draw a sprite, and then a background, it had to be time to put them together.
My first attempt at this didn’t work at all – all I got was the background, no sprite – until I learned that I need to convert my TileMap to a sprite also:
function initBg()
-- load spritesheet, and check it exists
bgSpriteSheet = gfx.imagetable.new("Images/bg-table-16-16.png")
assert( bgSpriteSheet )
-- define map
map = gfx.tilemap.new()
-- assign images
map:setImageTable(bgSpriteSheet)
-- def map size
map:setSize(15,15)
-- build output from myLevel, 15 blocks across
map:setTiles(myLevel, 15)
-- convert that map to a sprite for drawing
backgroundSprite = gfx.sprite.new(map) -- map to sprite
backgroundSprite:setCenter(0, 0)
backgroundSprite:moveTo(80, 0) -- center on screen
backgroundSprite:setZIndex(-1) -- behind player sprite
backgroundSprite:add()
end
.. and with that done (note setting the Z index) I could draw a sprite over the top, and have them both show.
But how about an animated one?
I drew up a quick ghost – four frames of 16×16:
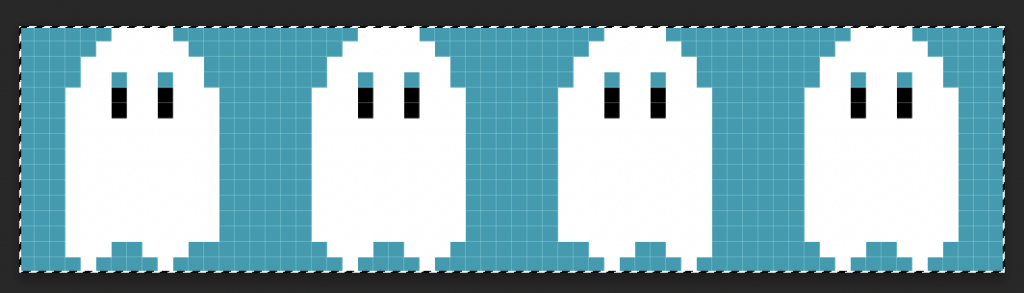
And then called in this library to make the job simpler. With that, I can initialise the ghost sprite, with animation:
function initGhost()
-- Set up the player sprite - animated ghosty
ghostTable = playdate.graphics.imagetable.new('Images/ghost')
assert( ghostTable )
playerSprite = AnimatedSprite.new(ghostTable)
playerSprite:playAnimation()
playerSprite:setCenter(0, 0)
playerSprite:moveTo( 96, 16 )
playerSprite:add()
end
.. and do some simple movement, just to test it, using playerSprite:moveBy.
The result:
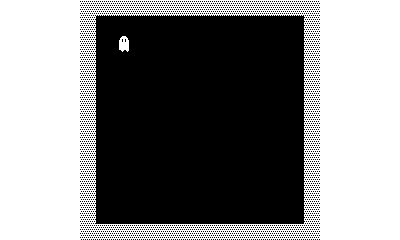
.. urgh, excuse the looping on that gif. But still, getting there!
Complete “moving ghosty an empty level” source here.