After a bit of a break to update some code for Make America Kittens Again and submit that to the Mac App Store, back to the little Pacman game.
Today: time to put the ghost back in motion. The key thing here is a function to check whether a given direction is a possible way to travel:
function checkForOpenPath(mapRefX,mapRefY,requestedDir)
-- calculate the grid ref targeted by the requested direction
-- then check if it is possible to move into that space
local targetRefX = mapRefX
local targetRefY = mapRefY
if requestedDir == "l" then
targetRefX = targetRefX - 1
elseif requestedDir == "r" then
targetRefX = targetRefX + 1
elseif requestedDir == "u" then
targetRefY = targetRefY - 1
elseif requestedDir == "d" then
targetRefY = targetRefY + 1
end
-- check for edge of grid
if (targetRefX < 1 or targetRefX > 15 or targetRefY < 1 or targetRefY > 15) then
-- target out of bounds
return false
end
-- check for wall
if map:getTileAtPosition(targetRefX,targetRefY) == 1 then
-- target is a wall
return false
end
return true
end
This gets called regularly to check for “ghost bumps into wall”, and also when the user tries to change direction.
The other things I learned: “not equals to” is checked using ~= rather than the != that I’m more used to, and I struggled to do a “is this value false” check, so need to look into that. For the moment I had to do this, which is clunky AF:
-- check for collision with wall on current path
if checkForOpenPath(mapRefX,mapRefY,playerDirection) then
-- nothing to see here
else
-- stop!
playerDirection = "n"
end
I also took out the timer as, at the moment, it just doesn’t seem needed.
And here’s the result:
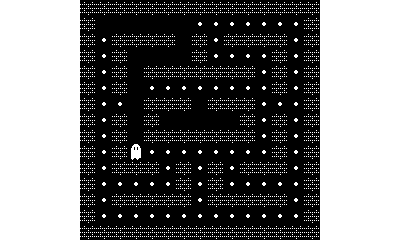
.. look at the little guy go. Full code here.