When all else fails and there’s nothing you can do: keep busy, I guess?
So, building on yesterday’s demo, I wanted to make my little ghost eat the dots as it passes over them. First step is working out when the ghost – which is moving in increments of 4px – has entered a new 16px unit of the map.
To test that, a helper function:
function isMultipleOf(testThis,divideBy)
if (testThis/divideBy == math.floor(testThis/divideBy)) then
return true
else
return false
end
end
And then when moving the ghost, use that to check:
if isMultipleOf(playerSprite.x - topLeft,16) then
if isMultipleOf(playerSprite.y,16) then
-- exactly on a square
checkForEat(((playerSprite.x-topLeft)/16)+1,(playerSprite.y/16)+1)
end
end
(Side note – I couldn’t get the code to compile when checking for both the isMultipleOf checks at once (if x AND y then). Could have just been a typo, need to check.)
In the call to checkForEat, we’re converting the pixel locations of the sprite (playerSprite.x, playerSprite.y) into a 1-indexed grid reference showing the sprite’s location in the 15 by 15 level – eg, x,y from 1,1 to 15,15.
We pass those to the function checkForEat, where we cast them to integers, and look up what tile is currently shown on that bit of the map:
function checkForEat(gridX,gridY)
-- check: is there a dot there? If so, we eat it
gridX = math.floor(gridX) -- cast to int in an ugly way
gridY = math.floor(gridY)
if map:getTileAtPosition(gridX,gridY) == 3 then
-- on a dot! chompy time
updateScore(100)
-- remove it
map:setTileAtPosition(gridX, gridY, 2)
end
end
If it’s 3 (my “dot” graphic), then we increase the score – via a function that will, later, draw the score somewhere too – and then update the tilemap to show a blank tile (tile 2) using setTileAtPosition.
So now as the ghost bobbles about, it eats the dots, and starts building a score:
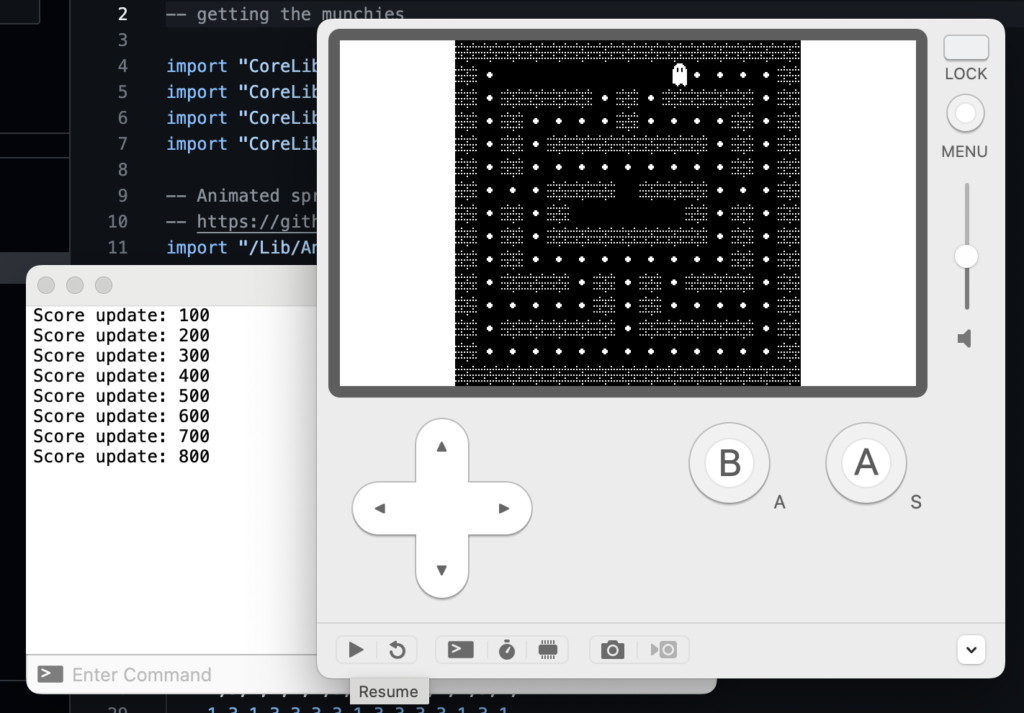
Full code here.